Deep Dive into React: Performance Optimization Strategies
Table of Contents
ToggleReact Speed Secrets: Keeping Your App Blazing Fast
Imagine this: you’ve poured your heart and soul into building an amazing React app. It’s got all the bells and whistles – stunning design, intuitive features, and powerful functionality. But then, disaster strikes! As users load your app, it feels sluggish, like wading through molasses. Yikes! A slow app can frustrate users and send them scrambling for the hills (or should we say, other tabs).
Fear not, React developer! This guide equips you with the knowledge and tools to keep your React app running at lightning speed. We’ll break down how React renders your app, uncover hidden performance bottlenecks, and share powerful techniques to make it feel like a race car, not a rusty clunker.
React’s Rendering Rhapsody: A Behind-the-Scenes Look
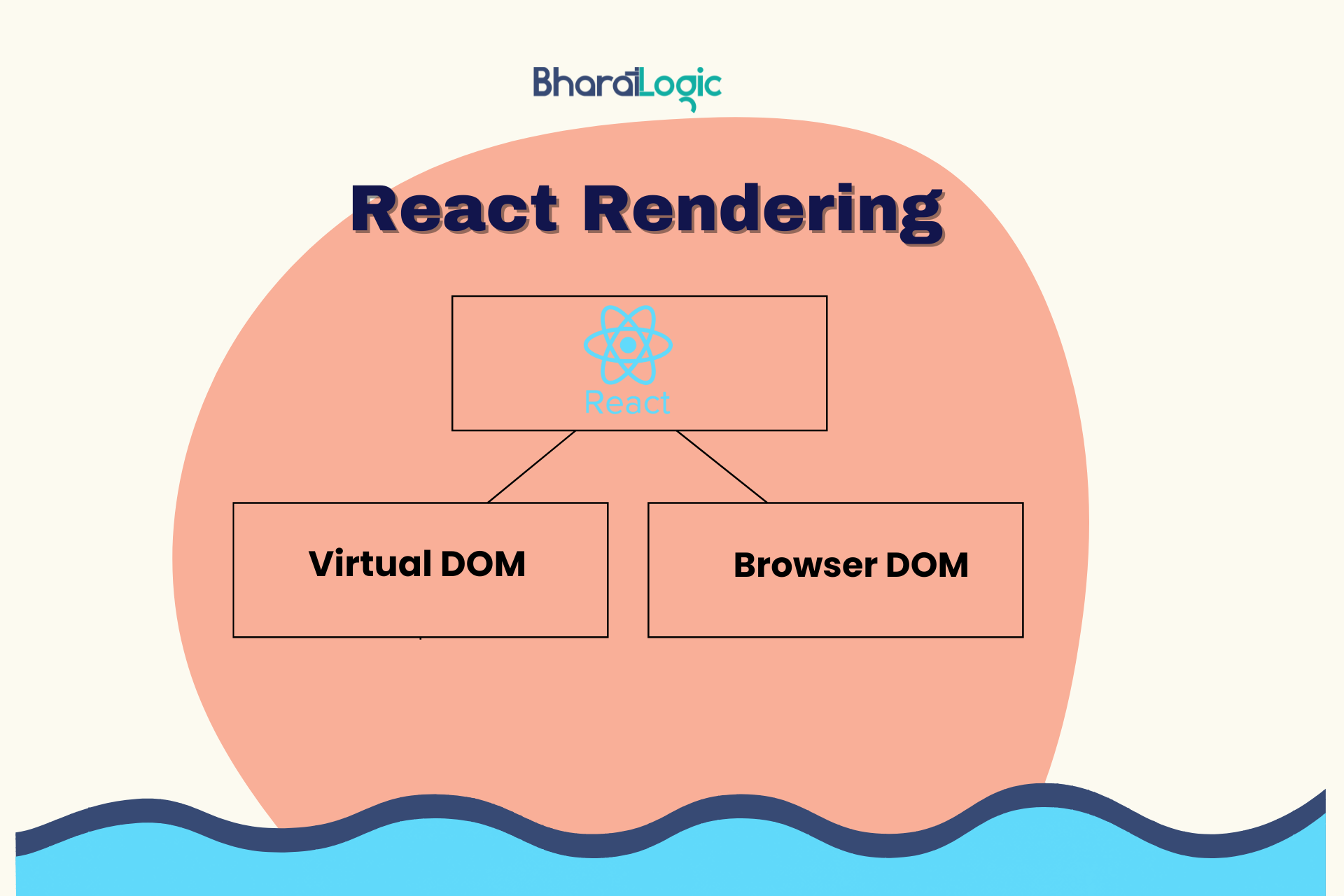
Let’s peek under the hood and see how React paints your beautiful app on the screen. Here’s the basic flow:
The Change Up: Whenever something in your app changes, like a button click or data update, React takes notice.
Virtual DOM Dance: React creates a lightweight, in-memory copy of your app’s structure called the virtual DOM. Think of it as a blueprint for the actual DOM (the real deal on the screen).
Diffing the Differences: React cleverly compares the new virtual DOM with the old one. It’s like finding the “what changed” in a game of “spot the difference.”
Real DOM Refresh: Based on the differences, React efficiently updates only the parts of the actual DOM that need changing. This keeps things smooth and avoids unnecessary rewrites.
While the virtual DOM is a performance champ, unnecessary re-renders can still slow things down. Here’s where our optimization journey begins!
Performance Culprits: The Obvious Suspects
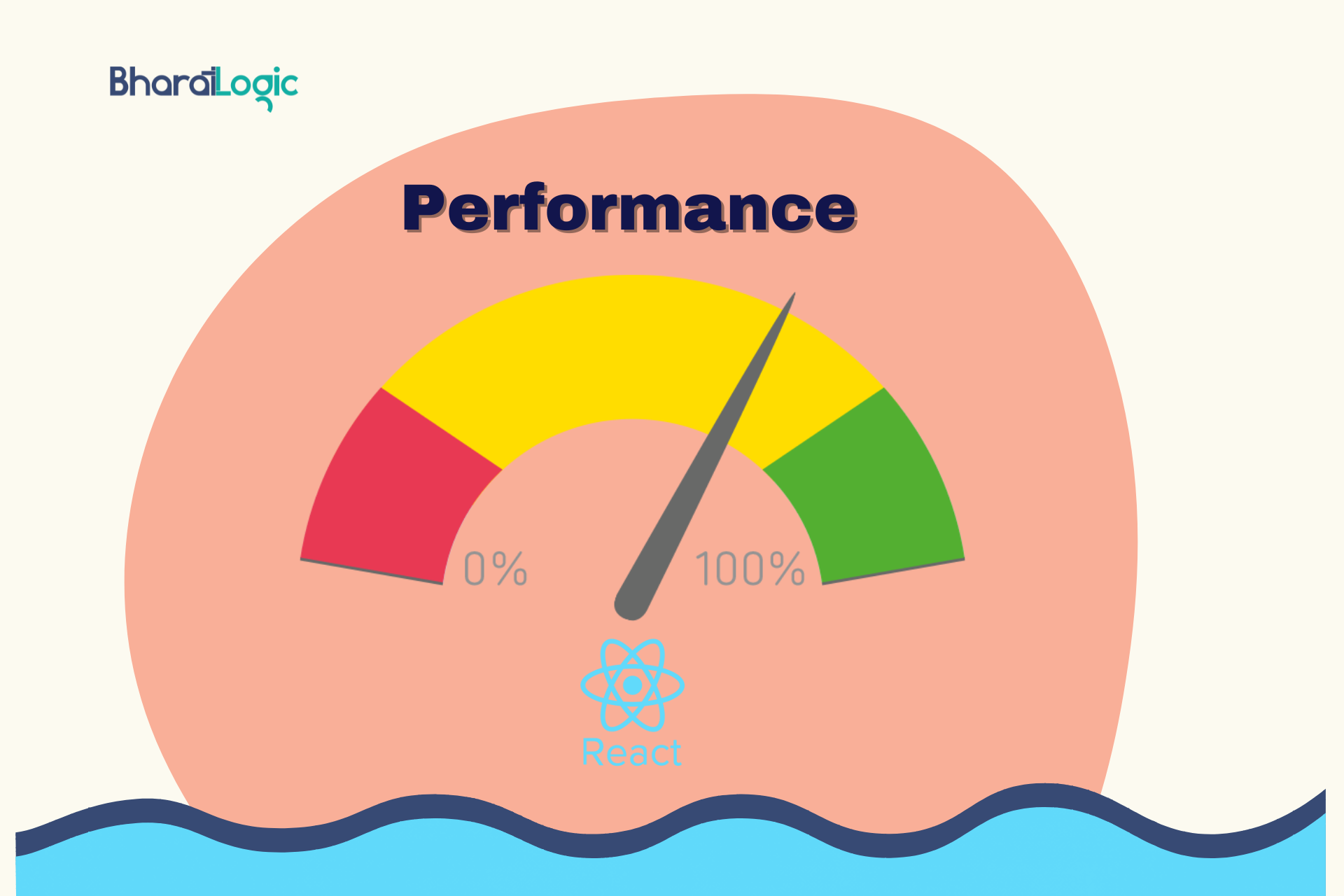
Let’s identify the common foes that can make your React app sluggish:
Re-render Rascals: When components re-render too often, even small changes can snowball into performance issues. Imagine a domino effect – one re-render triggers another, and another, and so on.
Render Heavyweight Champions: Packing heavy calculations or data fetching into render methods can slow down the rendering process. Think of it like trying to run a marathon while carrying a backpack full of bricks!
List Overload: Long lists of items, especially if not optimized, can overwhelm the browser and cause lag. Imagine a grocery list with hundreds of items – scrolling through it can feel like trying to find a needle in a haystack.
Library Landmines: Third-party libraries are great for adding functionality, but poorly chosen or implemented ones can drag down performance. Think of adding a fancy engine to your car, but it turns out to be super heavy and inefficient.
Now that we know the enemy, let’s arm ourselves with the weapons to defeat them!
Also: Some common issues and solutions on you MEAN stack app
React’s Built-in Performance Boosters
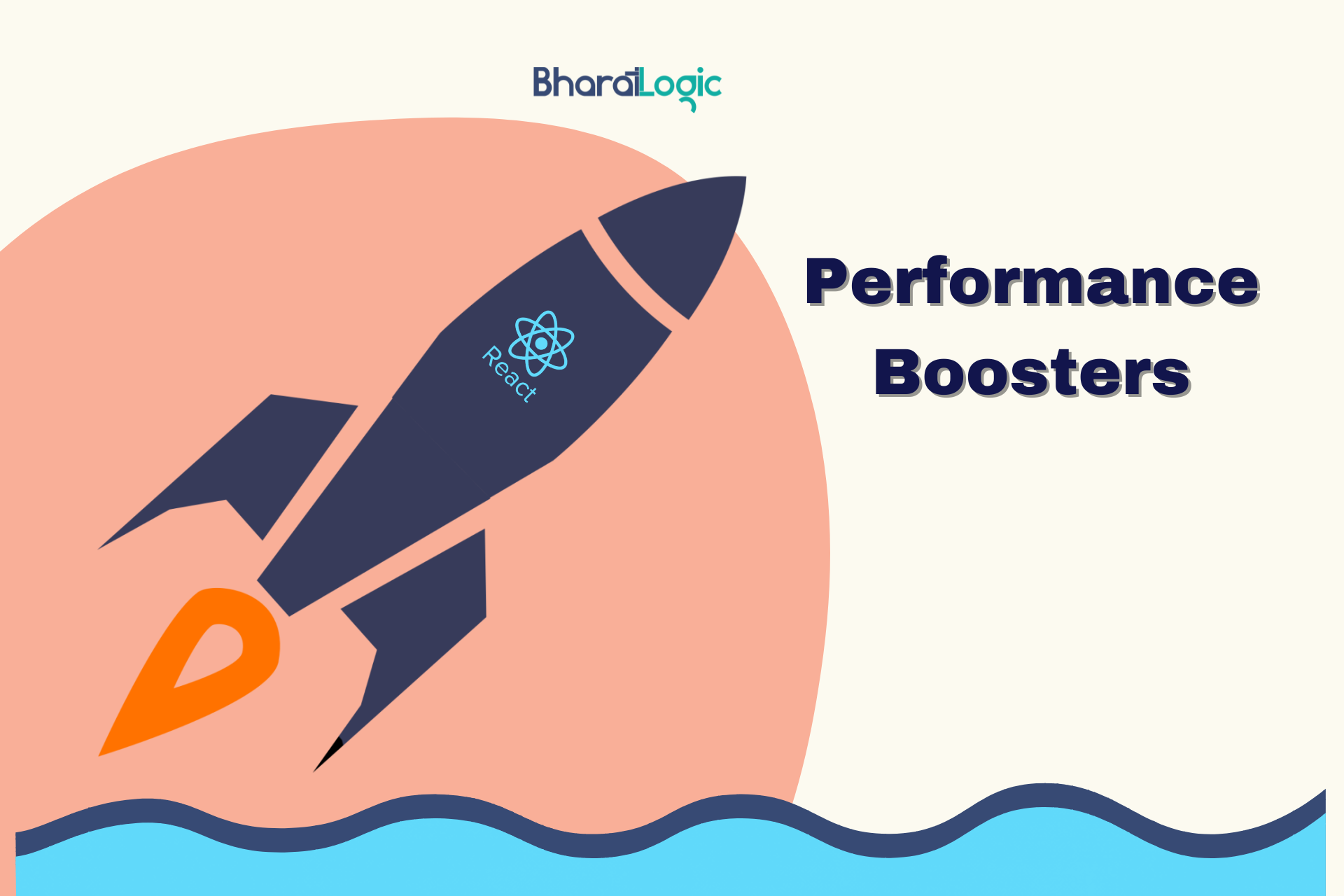
React comes with some built-in features to keep your app speedy:
PureComponent and React.memo: These are like bouncers for your components. They check if anything has truly changed before letting the component re-render, preventing unnecessary updates. Imagine a club with a strict “no-repeat-entry” policy unless something significant has changed about you.
ShouldComponentUpdate: This is your custom control panel. You can write code to decide exactly when a component should re-render, giving you fine-grained control over performance. Think of it like a dimmer switch for re-renders – you can adjust the level of strictness based on your needs.
useMemo and useCallback Hooks: Imagine a bakery that keeps fresh cookies ready. These hooks let you “memoize” expensive calculations or functions, meaning they’re only re-created if the ingredients (data) change. No need to waste time re-baking cookies if you already have a fresh batch!
Unleashing Performance: Techniques in Action
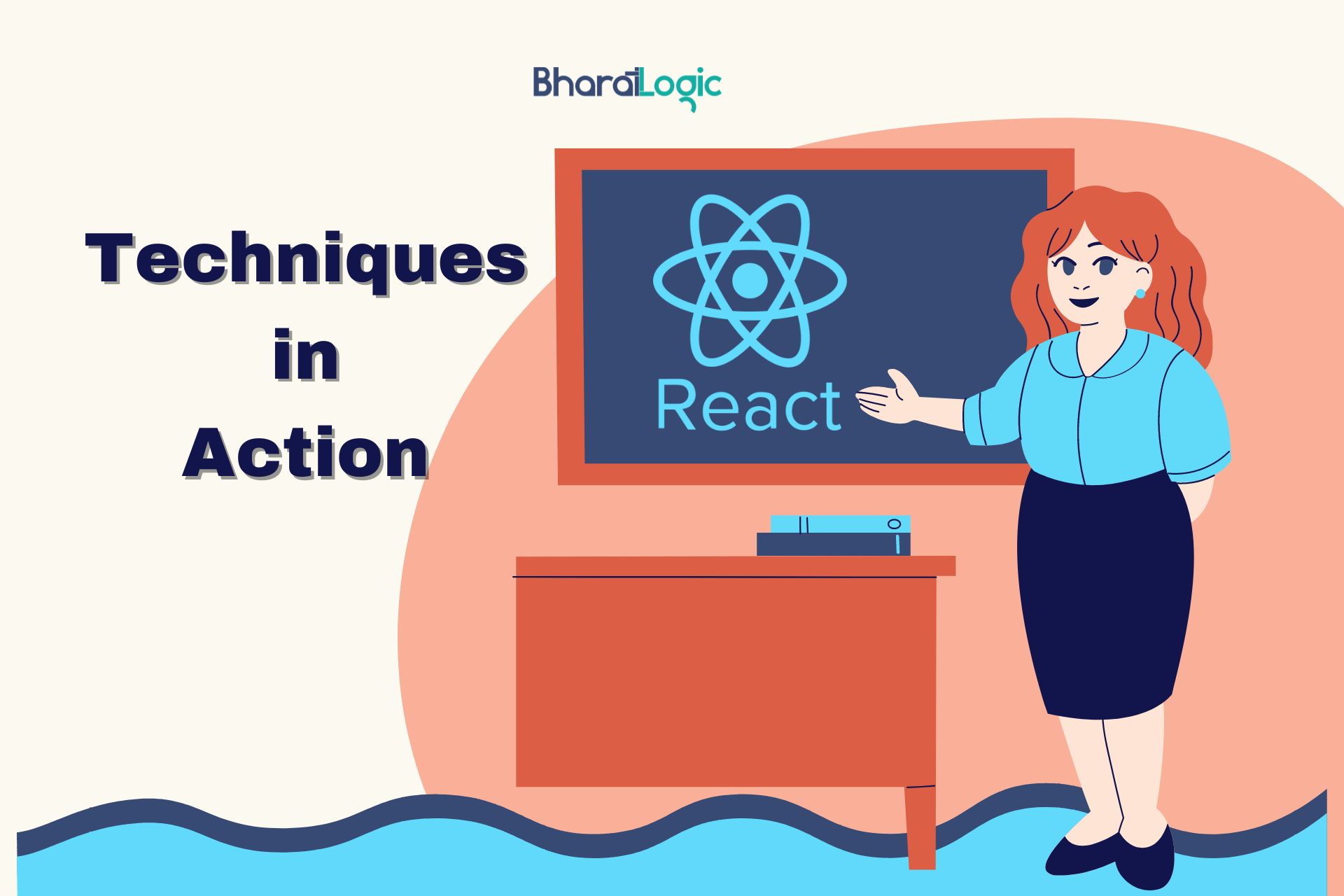
Let’s see these techniques in action, transforming your app from sluggish to super-fast:
1. Component Bootcamp:
Break Up the Big Guys: Large components can be overwhelming for React to render. Split them into smaller, more manageable ones for faster performance. Imagine chopping a large tree into firewood – it’s much easier to handle and burn smaller pieces!
Find the Pure Ones: Identify components that are truly “pure” (meaning they don’t have side effects like changing data outside the component). Use PureComponent or React.memo for them to prevent unnecessary re-renders. If a component is like a pure mathematical function, always giving the same output for the same input, these can be a big help.
Stop Prop Drilling: Imagine whispering a secret through a long line of people. Prop drilling, passing data through many levels
Stop Prop Drilling (Continued): of components, can be inefficient. Consider lifting state up or using context for shared data. Think of using a walkie-talkie instead of shouting through a line – it gets the message across more directly.
2. Rendering on Autopilot:
Lazy Load When Needed: Not everything needs to load at once. Use React. lazy and Suspense to load components only when they’re visible on the screen, like hidden features or tabs. Imagine a car that only loads the spare tire when you get a flat – no need to carry extra weight around if you don’t need it.
Render Only When Necessary: Conditionally render components based on state or props. If something isn’t needed, don’t waste time rendering it! Think of turning off lights in rooms you’re not using – conserve energy and improve performance.
Memoize the Heavy Lifters: Remember those bakery cookies? Use useMemo or useCallback to store the results of expensive calculations or functions and avoid re-doing them on every render. No need to re-calculate complex math problems if you already have the answer on hand.
3. Data Like a Boss:
Immutable Data Structures: Data that never changes is like a rock – solid and reliable. Consider using libraries like Immer to create immutable data structures. This forces React to only re-render the parts that actually changed, like updating a single brick in a wall instead of rebuilding the entire wall.
Virtualize Your Lists: Long lists can be a performance nightmare. Use libraries like React Virtualized or react-window. These render only the visible list items on the screen, keeping things smooth even with massive datasets. Imagine a grocery store that only displays the items on the current shelf you’re looking at, not the entire inventory at once.
4. Taming Third-Party Libraries:
Choose Wisely: Not all libraries are created equal. Evaluate third-party libraries for performance impact before adding them to your project. Consider alternatives if some are known to be heavy or poorly optimized. Think of adding tools to your toolbox – choose lightweight and efficient tools for the job.
Lazy Load When Possible: Similar to lazy loading components, you can leverage dynamic imports to load third-party libraries only when needed. This reduces initial load time. Imagine packing light for a trip – only bring what you’ll need at your destination.
5. Performance Detective Tools:
React Profiler: This built-in tool is your magnifying glass to identify components that are slowing down your app’s rendering. Think of it as a performance detective kit – it helps you pinpoint the culprits.
Browser DevTools: Your browser’s developer tools offer a wealth of information. These are like diagnostic tools for your app – they help you identify and fix problems.
Third-Party Performance Buddies: Consider libraries like why-did-you-render. These can help debug unnecessary re-renders and identify areas for optimization. Imagine having a performance consultant who helps you streamline your app.
The Final Lap: Keeping Your React App Speedy
Optimizing React application performance is an ongoing process. As your app grows and evolves, revisit these techniques and stay vigilant. Here are some additional tips:
Measure and Monitor: Regularly measure your app’s performance using the tools mentioned earlier. This helps you identify and address any performance regressions that might creep in over time. Think of it like regularly checking your car’s performance to ensure it’s running smoothly.
Stay Updated: Keep yourself updated with the latest React features and optimization best practices. The React community is constantly evolving, and new tools and techniques emerge frequently. Think of it like staying up-to-date on the latest car maintenance techniques.
Test Thoroughly: Performance testing should be an integral part of your development process. Test your app on different devices and network conditions to ensure a smooth experience for all users. Imagine test driving your car on different terrains to ensure it handles all conditions well.
By following these strategies and keeping performance optimization at the forefront of your development process, you can ensure that your React app stays fast, responsive, and delightful for your users. Remember, a happy user is a loyal user, and a fast app is a happy user!
If this blog post gives you clarity about these two JavaScript frameworks and is looking for the right expert to help you in your journey, Bharatlogic is the name you can easily trust and rely on for your projects. Bharatlogic is a leading expert in web and mobile app development. Backed by experienced programmers & developers, Bharatlogic is the right digital partner.