Debugging Your MEAN Stack Application: Common Issues and Solutions
The MEAN stack, with its power and flexibility, is a developer’s dream for building dynamic web applications. But even the dreamiest landscapes can have hidden thorns. When your MEAN stack application throws an error, frustration can quickly set in. Fear not, fellow developers! This guide equips you with the knowledge and tools to tackle common MEAN stack bugs and emerge victorious.
We’ll delve into the four pillars of the MEAN stack – MongoDB, Express.js, Angular, and Node.js – and explore their potential trouble spots. By understanding these areas, you’ll be well on your way to becoming a MEAN stack debugging champion.
Gird Your Loins for Battle: Essential Debugging Tools
Before we dive into specific errors, let’s arm ourselves with some essential tools for debugging:
Logging: Your best friend in the debugging world. Use console.log statements liberally in your Node.js code and browser console logs for Angular to track variable values and program flow.
Debuggers: Leverage built-in debuggers in your code editor (like VS Code) or browser developer tools to step through code line by line, inspect variables, and set breakpoints to pause execution at specific points.
Version Control (Git): A lifesaver for tracking changes and reverting to previous working versions if needed.
MongoDB: Taming the NoSQL Beast
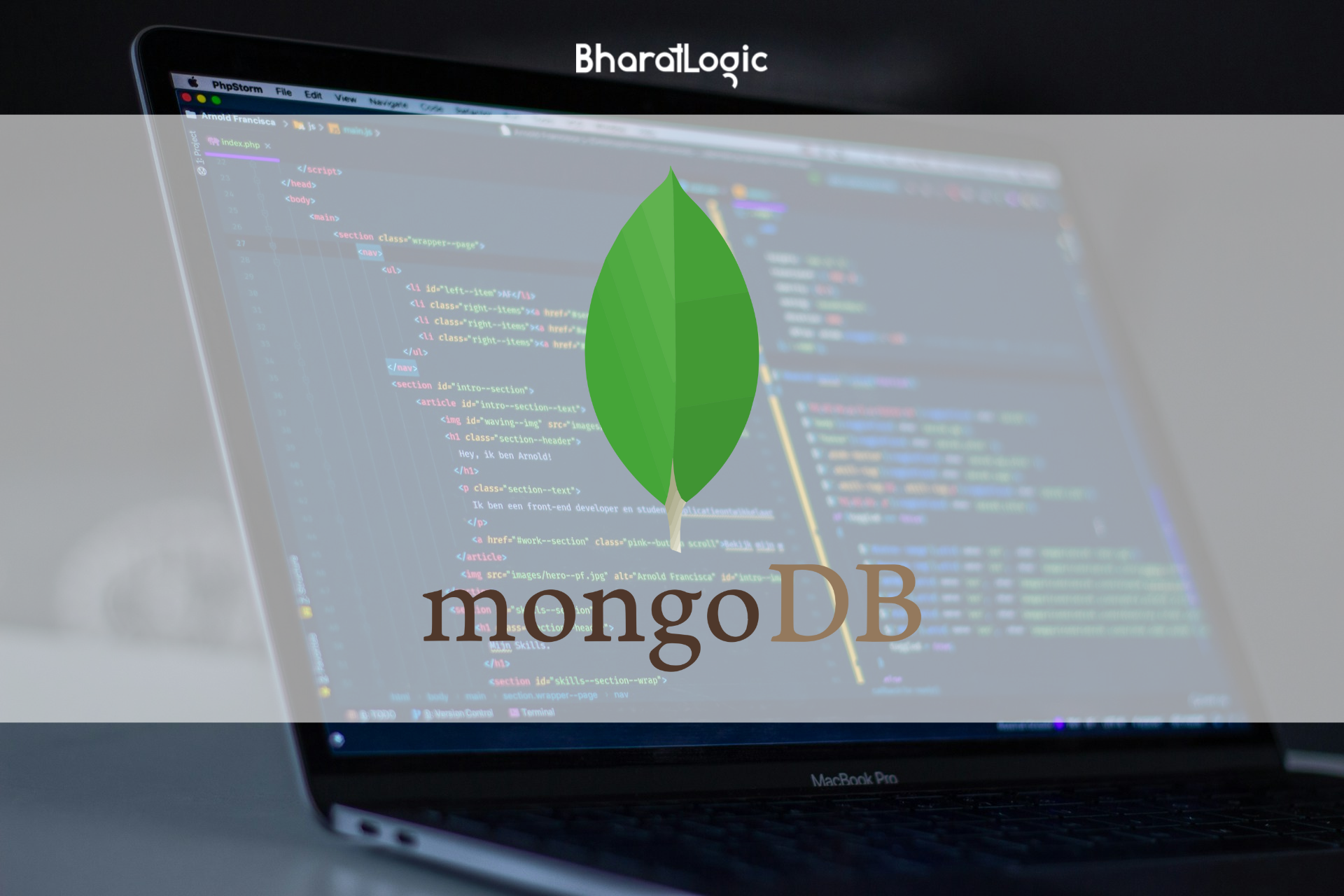
MongoDB, your chosen NoSQL database, might be the culprit for some errors.
Connection Errors: Ensure your MongoDB connection string is correct in your Node.js code. Verify that MongoDB is running and accessible.
Incorrect Queries: Double-check your Mongoose queries for syntax errors and logical mistakes. Use tools like Robo 3T or MongoDB Compass to test queries directly in the database.
Data Validation Issues: Implement Mongoose schemas to enforce data types and required fields, preventing invalid data from entering the database.
Express.js: Wrangling the Server-Side Dragon
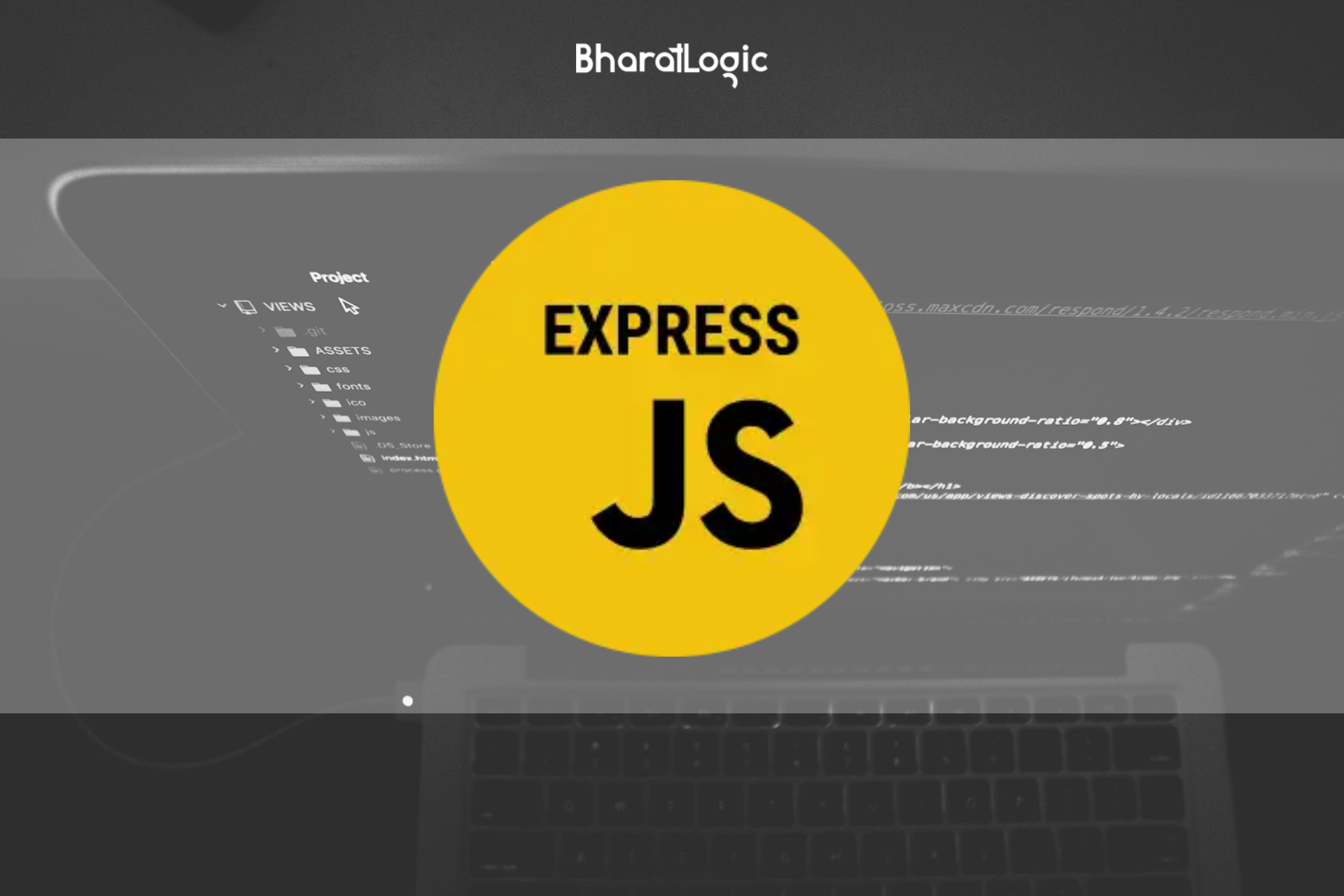
Express.js handles your application’s backend logic. Here’s how to tame potential issues:
Middleware Mayhem: Middleware functions can chain together and cause unexpected behavior. Use console.log statements within middleware to track request and response objects and pinpoint the source of the problem.
Routing Woes: Check for typos in your route definitions and ensure the correct HTTP method (GET, POST, etc.) is used for each route.
Error Handling: Implement proper error handling using try-catch blocks to capture and log errors gracefully, providing more informative messages for debugging.
Angular: Mastering the JavaScript Framework
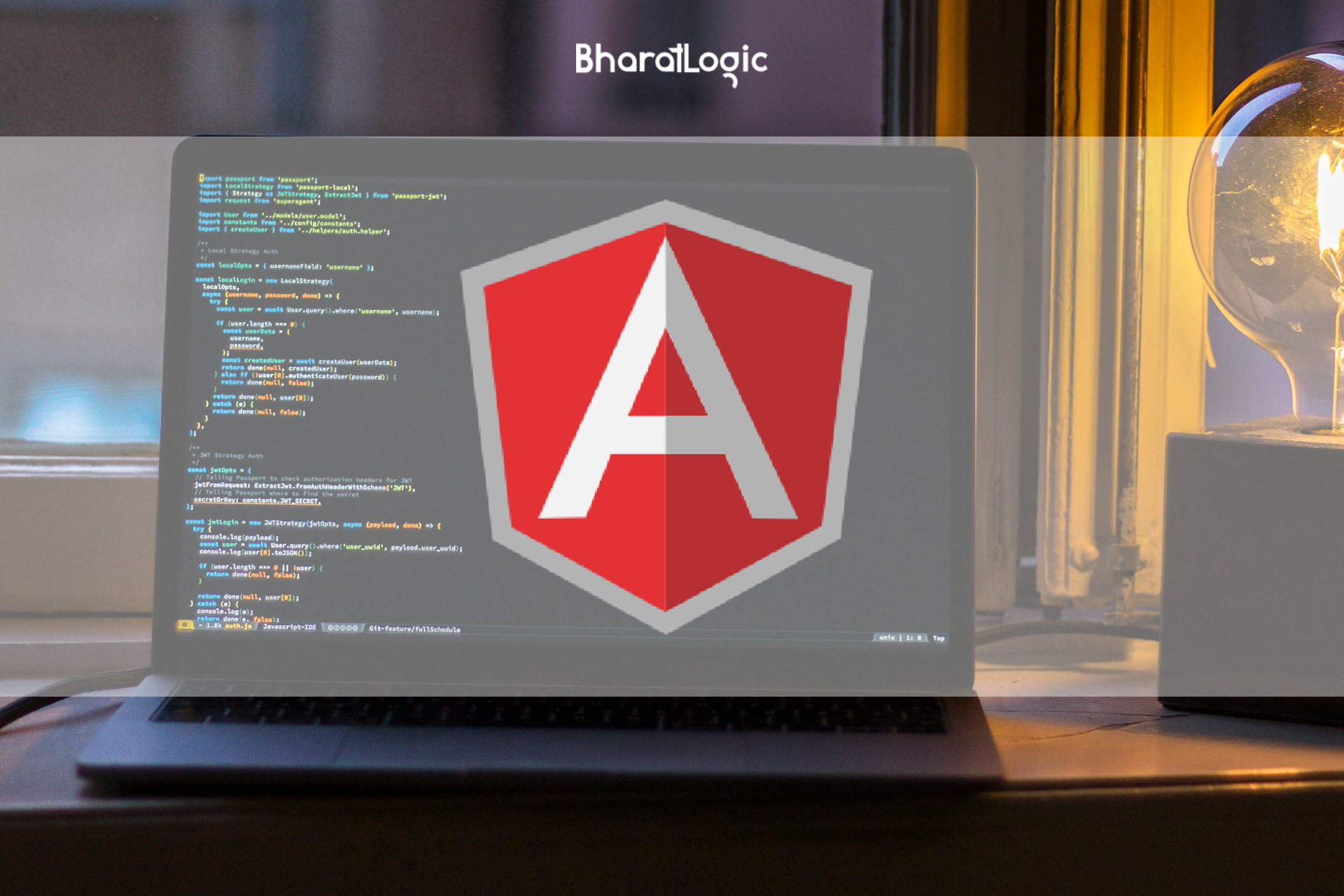
Angular, your front-end champion, can sometimes throw curveballs:
Component Communication: Ensure proper communication between components using services, event emitters, or input/output bindings. Debugging tools like the Angular CLI’s ng generate component my-component –test can help isolate component-specific issues.
Template Syntax Errors: Typos or syntax errors in your Angular templates can lead to unexpected behavior. Utilize browser developer tools to identify these issues and their affected components.
Dependency Injection Mishaps: If components or services rely on injected dependencies, verify they are correctly provided and configured in your module providers.
Node.js: Taming the JavaScript Runtime
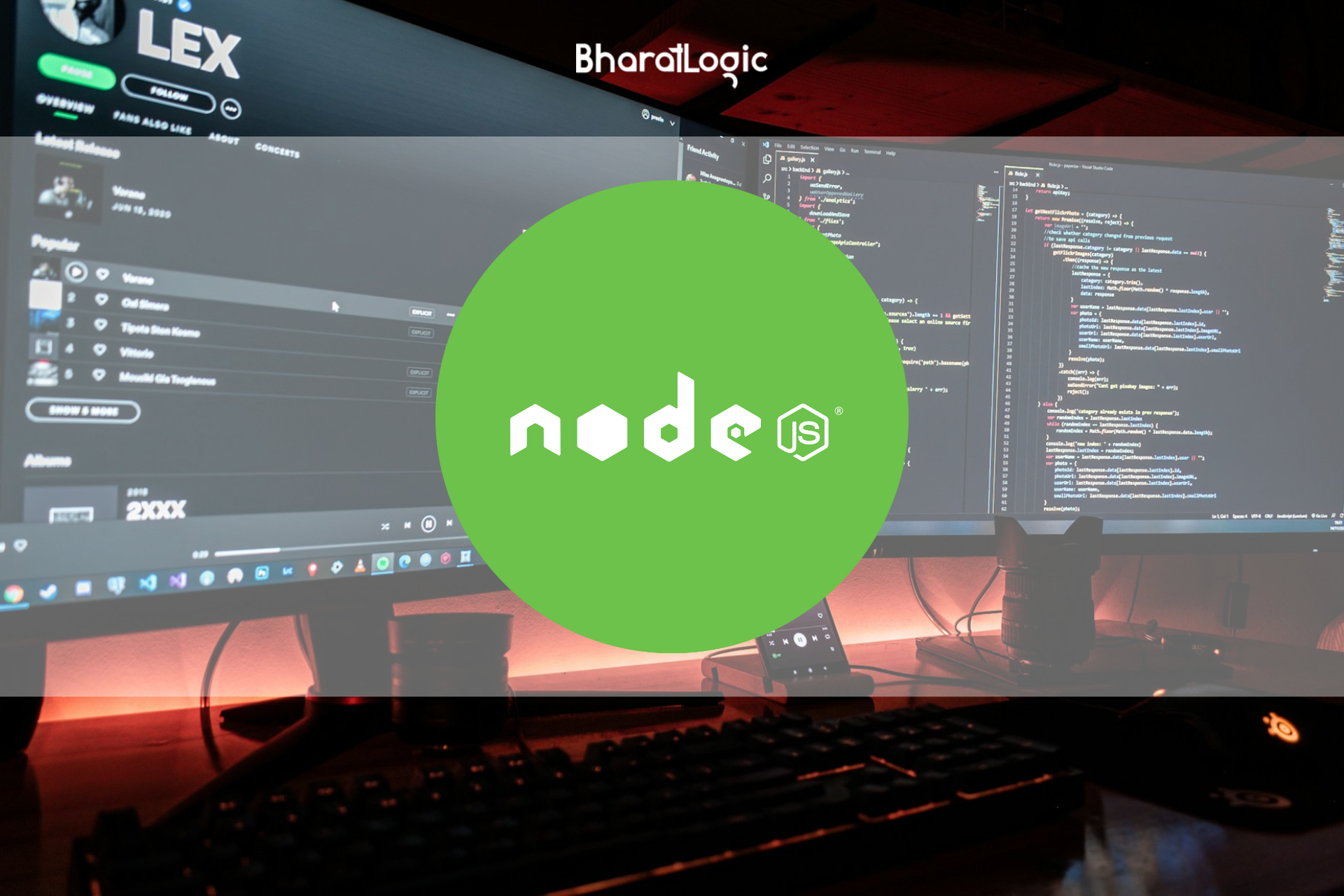
Node.js, the engine powering your application, can also be a source of errors:
Asynchronous Nightmares: Asynchronous code using callbacks or promises can get messy. Use debugging tools to track the execution flow and identify potential race conditions or unhandled rejections.
Module Resolution Issues: Ensure your project’s directory structure is organized correctly, and dependencies are properly installed and referenced in your package.json file.
Server Crashes: If your server crashes mysteriously, check server logs for error messages. Common culprits include unhandled exceptions, memory leaks, or resource exhaustion. Utilize tools like PM2 for process management and health monitoring.
Beyond the Basics: Advanced Debugging Techniques
Once you’ve mastered the fundamentals, consider these advanced techniques:
Debugging in Production: Configure your application to log errors more verbosely in production environments while still maintaining security. Tools like Sentry can provide detailed error reports from production deployments.
Unit Testing: Implementing unit tests for your application components helps catch errors early in the development process. Frameworks like Jest or Mocha can streamline this process.
Static Code Analysis: Tools like ESLint can analyze your code for potential issues like syntax errors, unused variables, and code smells before they cause runtime problems.
Remember: Debugging is an iterative process. Don’t get discouraged if you don’t find the solution immediately. Be methodical, use your tools effectively, and leverage online resources and communities when needed Conquering
Specific Error Messages: Examples and Solutions
Now that we’ve explored common pitfalls in each layer of the MEAN stack, let’s delve into specific error messages you might encounter and their potential solutions:
MongoDB Errors:
“CastError: The provided value…”: This error indicates a type mismatch. Ensure you’re inserting data that adheres to your Mongoose schema definitions.
“MongoNetworkError: Could not connect to server…”: Double-check your connection string and verify that MongoDB is running and accessible on the specified host and port.
“MongoError: write to {collection name} failed…”: This could signify a validation error. Check your Mongoose schema for required fields or data type constraints.
Express.js Errors:
“Cannot GET /your-route”: Verify the route definition in your Express app matches the requested URL and HTTP method (GET, POST, etc.).
“TypeError: next is not a function”: This indicates an issue with middleware execution flow. Ensure you’re calling next() within your middleware functions to continue the request processing chain.
“500 Internal Server Error”: This generic error often points to unhandled exceptions within your routes or middleware. Utilize try-catch blocks to capture and log errors for further investigation.
Angular Errors:
“Error: NgModel cannot be used…”: Common in forms, this error usually indicates a missing or incorrect form control name in your template.
“TypeError: Cannot read property ‘…’ of undefined”: This points to an attempt to access a property on an object that might be undefined. Use conditional checks to handle scenarios where the object might not be present.
Node.js Errors:
“TypeError: Cannot read property ‘…’ of undefined”: Similar to Angular, this error signifies accessing properties on undefined objects in your Node.js code. Utilize checks like if (object && object.property) for safe access.
“ReferenceError: variable is not defined”: Ensure variables are declared and assigned values before using them. Check for typos in variable names.
“Module not found…”: Verify the module you’re trying to import exists in your project directory or is installed as a dependency using npm install.
Remember: These are just a few examples, and error messages can vary depending on the specific libraries and frameworks you’re using. However, understanding the underlying concepts behind these errors will equip you to tackle a broader range of issues.
Also : Explore the top 5 React development companies in USA
Beyond Debugging: Proactive Measures for a Smoother Journey
While debugging is a crucial skill, here are some proactive measures to minimize the need for reactive firefighting:
Write Clean Code: Follow coding best practices like clear variable naming, proper indentation, and modular design. Clean code is easier to understand and maintain, leading to fewer bugs.
Utilize Comments: Document your code with clear comments explaining the purpose of functions and complex logic. This will save you time when revisiting your code in the future and help teammates understand your thought process.
Test Early and Often: Implement unit tests for your components and services. Utilize tools like Jest or Mocha to run these tests automatically and catch errors early in the development cycle.
Code Reviews: Conduct code reviews with your team. This allows for peer-to-peer learning and can help identify potential issues before they become major problems.
Stay Updated: Keep your development tools, libraries, and frameworks updated with the latest versions to benefit from bug fixes and security patches.
Conclusion: Debugging Like a MEAN Stack Master
By understanding common pitfalls in each layer of the MEAN stack, equipping yourself with the right tools and techniques, and adopting a proactive approach to code quality, you’ll transform from a frustrated developer to a MEAN stack debugging champion. Remember, patience, persistence, and a dash of creativity are your allies in this battle against bugs. So, the next time your application throws an error, take a deep breath, grab your debugging tools, and approach the challenge with a problem-solving mindset. You’ve got this!
Numerous frontend frameworks are available in the market for web development. Picking up the right one seems like finding a needle in a haystack. But we are sure kicking off your hunt keeping in mind these factors will help you end your search soon. If you want an expert opinion, you can also get in touch with professionals of Bharatlogic– a leading web development company in the USA. Backed by 100+ tech experts, Bharatlogic is a renowned name in the digital world that helps you accomplish your goals with quality output.